Choosing the right tool for building websites is important. Svelte and React are two popular options, each with its own strengths. React has been around for a while and is widely used, while Svelte is newer but gaining popularity quickly.
In this blog, we’ll compare Svelte and React in simple terms. We’ll look at how they work, how easy they are to learn, how fast they are, and the community support around them. Whether you’re new to web development or an experienced coder, this guide will help you decide which one is better for your projects. Let’s dive in and see which one comes out on top!
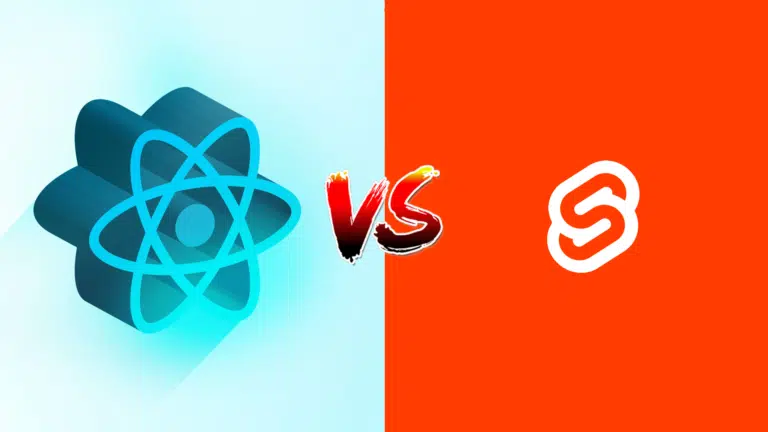
What is React?
React, also known as React.js or ReactJS, is a JavaScript library for building user interfaces. It was developed by Facebook (now Meta) and is maintained by Facebook and a community of individual developers and companies. React is used for handling the view layer for web and mobile apps, allowing developers to create reusable UI components.
Key Features of React
- Virtual DOM (Document Object Model): React employs a virtual DOM to improve performance. The virtual DOM is a lightweight copy of the actual DOM. React keeps track of changes in the virtual DOM and efficiently updates only those parts of the real DOM that have changed, leading to faster rendering.
- JSX (JavaScript XML): React uses JSX, a syntax extension for JavaScript. JSX allows you to write HTML structures in the same file as your JavaScript code. This makes the code more readable and easier to debug.
- Declarative UI: In React, you describe the UI declaratively. When the data changes, React automatically manages the updates to the DOM, ensuring that the UI is always in sync with the state of the application.
- One-Way Data Binding: React follows a unidirectional data flow, meaning that the data in React has a single flow from parent to child components. This approach makes the data flow easier to understand and debug.
- Hooks: Introduced in React 16.8, hooks are functions that let you “hook into” React state and lifecycle features from function components. They provide a more straightforward API for managing state and side effects in components.
- Strong Community and Ecosystem: React has a vast and active community, offering a plethora of libraries, tools, and extensions. This ecosystem makes it easier for developers to find solutions to common problems and learn best practices.
React Performance
React is known for its performance, mainly due to its efficient update and rendering system.
- Virtual DOM: React’s use of the virtual DOM minimizes direct manipulation of the actual DOM, which is a costly operation in terms of performance. By only updating the parts of the DOM that have changed, React reduces the time and resources required for updates.
- Component Lifecycle Methods: React provides lifecycle methods that allow developers to control and optimize the behavior of components throughout their lifecycle, from mounting to updating to unmounting.
- ShouldComponentUpdate and React.memo: These are methods and higher-order components provided by React to prevent unnecessary renders. Developers can control component re-rendering to improve performance, especially in cases of complex applications.
- Hooks for Optimization: The
useMemo
anduseCallback
hooks help to avoid unnecessary computations and re-renders, respectively, by memorizing values and functions. - Concurrent Mode: An experimental feature in React, Concurrent Mode, allows for interruptible rendering, enabling the UI to stay responsive during heavy rendering tasks.
- Server-Side Rendering (SSR): React can render components on the server and send them as HTML to the client. SSR improves the initial load time and SEO, as the content is readily available to search engines.
- Code Splitting: React supports code splitting out of the box with
React.lazy
andSuspense
. This feature allows you to split your code into smaller chunks which are loaded on demand, thus reducing the initial load time.
Pros of React
Large Ecosystem and Community: React has a vast and active community, providing an extensive range of libraries, tools, tutorials, and community support. This ecosystem is invaluable for developers, offering solutions for almost any challenge.
Component-Based Architecture: React’s modular approach allows for reusable components, making code more manageable and scalable. It promotes cleaner code and easier maintenance.
Virtual DOM for Enhanced Performance: React’s Virtual DOM improves application performance, especially in complex applications. It minimizes direct manipulation of the actual DOM, which is costly in terms of resources.
Strong Backing by Facebook (Meta): React is maintained by Facebook, ensuring regular updates, a roadmap for future development, and stability for enterprise applications.
JSX (JavaScript XML): JSX simplifies the process of writing components and makes the code more readable. It allows for HTML-like syntax directly in JavaScript code.
Strong Job Market: React developers are in high demand across the technology industry, making it a beneficial skill for career growth.
Flexibility and Compatibility: React can be used in a variety of projects, from small personal websites to large-scale enterprise applications. It’s also compatible with other libraries and frameworks.
Server-Side Rendering (SSR): React’s ability to render on the server side is beneficial for SEO and initial page load performance.
Cons of React
Steep Learning Curve: React’s ecosystem, including Redux for state management and routing libraries, can be overwhelming for beginners. JSX and advanced concepts like hooks also require time to learn.
Rapid Pace of Development: The frequent updates and changes in React and its ecosystem can be challenging to keep up with, requiring developers to continuously learn and adapt.
Boilerplate Code: Larger applications in React can end up having a lot of boilerplate code, which can make the project complex and harder to manage.
Performance Overheads in Complex Apps: For very complex applications, performance issues can arise, particularly with unnecessary re-renders and large component trees.
SEO Challenges: Single Page Applications (SPAs) built with React can face challenges with search engine optimization, although this can be mitigated with SSR and other strategies.
Proprietary Syntax (JSX): JSX, while beneficial, is a proprietary syntax that blends HTML and JavaScript, which some developers may not prefer.
Overreliance on Third-Party Libraries: React often requires additional libraries for state management, routing, etc., which can lead to dependency on external solutions and a fragmented architecture.
What is Svelte?
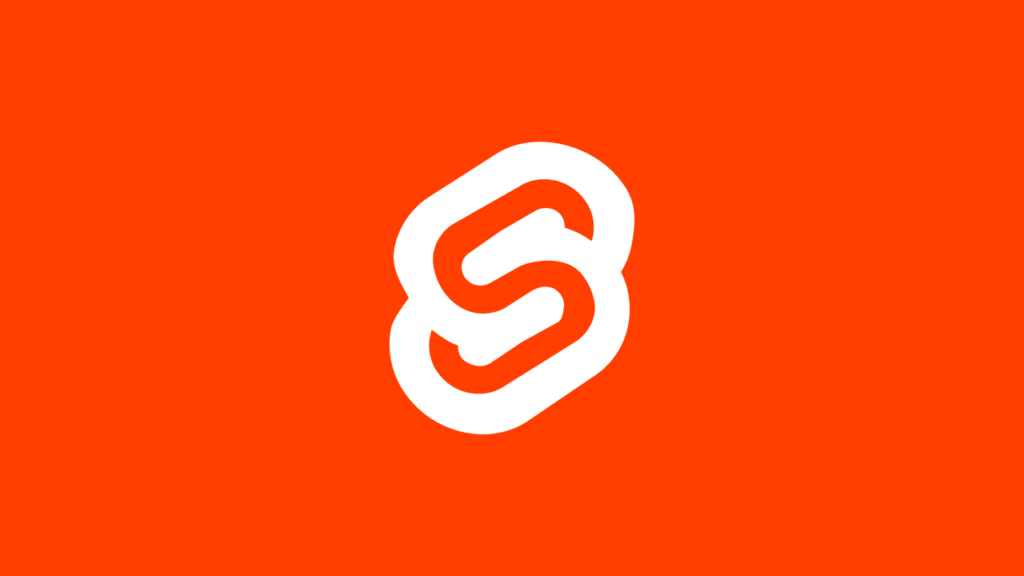
Svelte is an innovative framework for building user interfaces. Unlike traditional frameworks like React or Vue, Svelte shifts much of the work to compile time, producing highly optimized vanilla JavaScript at the end. This unique approach sets it apart in the modern web development landscape. It was created by Rich Harris and has been gaining popularity for its simplicity and performance advantages.
Key Features of Svelte
- Compile-Time Framework: Unlike frameworks that do most of their work in the browser at runtime, Svelte shifts that work into a compile step that happens when you build your app. This results in smaller, more efficient JavaScript code.
- No Virtual DOM: Svelte does away with the Virtual DOM, a feature commonly used in other frameworks. Instead, it updates the DOM when the state of the app changes, which can lead to better performance.
- Reactive Programming Model: Svelte introduces a simpler reactive programming model. You write plain JavaScript, and the compiler figures out the minimal set of DOM updates needed when your data changes.
- Component-Based Architecture: Like React, Svelte is also component-based. Each component is a self-contained unit with its own state and behavior, making them easy to reuse and manage.
- Less Boilerplate Code: Svelte requires significantly less boilerplate, leading to more concise and readable code. This is largely due to its reactive nature and the compiler doing much of the heavy lifting.
- Built-in State Management: Svelte has a straightforward way to handle state management within components, eliminating the need for external libraries for state management in many cases.
- Rich Motion and Transition Support: Svelte provides built-in utilities for animations and transitions, making it easy to implement complex UI animations.
- Small Bundle Sizes: Due to its compile-time approach, the resulting JavaScript bundles are typically smaller, which is beneficial for performance, especially on mobile devices.
Svelte Reactivity
Svelte’s approach to reactivity is one of its most defining features, and it’s remarkably straightforward:
- Assignment-Based Reactivity: In Svelte, the reactivity is triggered by assignments. When you assign a new value to a variable, Svelte automatically updates the DOM to reflect the new state.
- Reactive Declarations: You can create reactive declarations using a simple
$:
syntax. This tells Svelte to rerun the code whenever any of the values in the reactive statement change. - Reactive Statements: These are simple JavaScript statements that automatically update when their dependencies change. This eliminates the need for a complex API to handle reactivity, as seen in other frameworks.
- Efficient DOM Updates: Since Svelte compiles to vanilla JavaScript and updates the DOM directly without the need for a virtual DOM, the updates are typically very efficient.
- Reactivity with Stores: For global state management, Svelte introduces the concept of stores. A store is a reactive object that components can subscribe to and receive updates from, providing a simple and effective way to manage shared state.
Pros of Svelte
Compile-Time Optimization: Svelte does much of its work at compile time, translating your components into highly optimized JavaScript, which can lead to better performance and smaller bundle sizes.
Simplified Code: Svelte’s syntax is straightforward and requires less boilerplate than many other frameworks. This makes your codebase cleaner and more readable.
No Virtual DOM: Unlike frameworks that use a Virtual DOM, Svelte writes code that updates the DOM when the state of your application changes. This can result in more efficient updates and better performance, particularly for frequent, small updates.
Reactive by Design: Svelte’s reactivity model is simple yet powerful. Reactivity is built into the language itself, and there’s no need to learn additional concepts like hooks or observables for basic reactivity.
Built-In Transition and Animation Support: Svelte comes with rich support for transitions and animations, making it easy to create interactive and visually appealing interfaces.
Ease of Learning: Thanks to its less complex and more intuitive syntax, Svelte can be easier to learn for those new to web development or coming from a non-JavaScript background.
Community and Ecosystem: While smaller than communities like React, Svelte’s community is growing and passionate, contributing to a growing ecosystem of tools and libraries.
Cons of Svelte
Smaller Community and Ecosystem: Compared to more established frameworks like React or Vue, Svelte’s community is smaller. This means fewer resources, tutorials, and third-party libraries.
Less Mature: Being a newer framework, Svelte might not have the same level of maturity as other frameworks, which can sometimes lead to encountering edge cases or bugs that have not been widely addressed yet.
Limited Job Market: The job market for Svelte developers is currently smaller than for more widely adopted frameworks like React or Angular, which could be a consideration for career-focused developers.
Less Plugin/Integration Support: Given its smaller ecosystem, you might find fewer plugins and integrations available for Svelte compared to other major frameworks.
Learning Curve for Advanced Features: While Svelte is easy to pick up, mastering its more advanced features and concepts, especially around stores and reactivity, can take time.
Potential Overhead in Large Applications: For very large applications, the lack of a Virtual DOM can sometimes lead to performance issues, as direct DOM manipulations can become costly.
Lack of Enterprise-Level Adoption: Svelte is not yet as widely adopted in large enterprises as some other frameworks, which can be a consideration for large-scale projects.
Similarities Between Svelte vs React
React and Svelte, despite their differences in design philosophy and implementation, share several similarities that are worth noting. These similarities often make them contenders for similar types of web development projects. Here are the key similarities:
- Component-Based Architecture: Both React and Svelte utilize a component-based architecture. This approach involves building applications as a composition of small, reusable components, each responsible for managing their own state and behavior.
- Declarative Approach to UI: React and Svelte both allow developers to describe UIs declaratively. In React, this is done using JSX, while Svelte uses a template syntax that resembles HTML. In both cases, you describe what the UI should look like, and the framework takes care of rendering it based on the current state.
- Client-Side Rendering: Both frameworks are primarily used for building single-page applications (SPAs) where the bulk of the rendering work happens in the user’s browser, allowing for dynamic and interactive user experiences.
- Reactivity and State Management: React and Svelte offer ways to manage the application state and respond to changes in that state. React does this through hooks and state management libraries like Redux, while Svelte has built-in reactivity that automatically updates the UI when state changes.
- JavaScript Ecosystem: As JavaScript frameworks, both React and Svelte benefit from the broader JavaScript ecosystem, including NPM packages, tooling, and community resources. Developers can leverage a wide array of libraries and tools available in the JavaScript world.
- Focus on Performance: Both frameworks emphasize performance, albeit through different means. React uses the Virtual DOM to minimize direct DOM manipulation, while Svelte compiles away the framework itself, leading to fewer runtime overheads.
- Modern Web Development Features: React and Svelte support modern web development practices like server-side rendering, code splitting, and integration with various build tools and preprocessors.
- Community and Resources: Both have active communities, providing resources like documentation, tutorials, community support, and third-party libraries, although React’s community is significantly larger.
Key Differences Between React and Svelte
1. Underlying Philosophy and Approach
The underlying philosophy and approach of React and Svelte indeed highlight the fundamental differences in how they handle web development tasks:
React
- Library vs. Framework: React is more accurately described as a JavaScript library rather than a full-fledged framework. It’s specifically focused on the UI layer, leaving other aspects like routing or state management to be handled by additional libraries or frameworks.
- Runtime-Based: React’s operations are largely runtime-based. When you build a React app, the final product still relies on the React library to manage the UI, requiring the browser to execute a significant amount of JavaScript.
- Explicit Data Flow: React emphasizes explicit data flow through props (properties) and state. The idea is to make the flow and manipulation of data within the application as transparent and predictable as possible, often leading to more manageable code, especially in large applications.
- Emphasis on Immutability and Functional Programming: React encourages the use of immutable data structures and functional programming concepts, which align with its declarative nature and help in predictable state management and UI rendering.
Svelte
- Compiler Approach: Svelte takes a unique approach by being a compiler. Instead of shipping a library that runs in the browser, Svelte compiles your code into small, optimized vanilla JavaScript at build time. This results in less JavaScript needing to be downloaded, parsed, and executed in the client.
- Direct DOM Manipulation: Svelte writes code that updates the DOM directly when the state changes. This approach differs significantly from Virtual DOM-based frameworks and can lead to more efficient updates, especially for smaller, frequent changes.
- Reactivity Baked into the Language: Svelte’s reactivity model is more straightforward and less verbose. It makes state management simpler by automatically re-rendering the appropriate component parts when their dependent state changes.
- Simplicity and Developer Experience: Svelte’s design philosophy prioritizes simplicity and ease of use. It aims to reduce boilerplate and provide a more intuitive development experience, making it accessible to a broader range of developers.
2. Virtual DOM vs. Direct DOM Manipulation
The comparison between React’s Virtual DOM and Svelte’s direct DOM manipulation approach is a key differentiator in how they manage UI updates:
React and Virtual DOM
- Virtual DOM Mechanism: React employs a Virtual DOM, which is an in-memory representation of the real DOM elements. It’s a lightweight copy that allows React to perform operations in an efficient and optimized way.
- Diffing Algorithm: When the state or props of a component change, React first updates this Virtual DOM. Then, it uses a diffing algorithm to compare the updated Virtual DOM with the previous version, identifying exactly which parts of the actual DOM need to be updated.
- Batched Updates for Efficiency: This process allows React to batch multiple changes into a single update cycle, minimizing direct manipulation of the real DOM, which is a performance-intensive task.
- Reduced Direct DOM Interaction: By minimizing direct interaction with the DOM, React can improve performance, especially in complex applications where frequent DOM updates would be costly.
Svelte and Direct DOM Manipulation
- Compiler Approach: Svelte, as a compiler, converts your components into highly optimized imperative JavaScript code. This code directly manipulates the DOM without the need for an intermediate representation like the Virtual DOM.
- Efficiency in Updates: When a state change occurs in Svelte, the compiled code updates the DOM directly and precisely where the change needs to be reflected. This eliminates the overhead associated with the Virtual DOM’s diffing and re-rendering process.
- Less Overhead in Runtime: By avoiding the Virtual DOM, Svelte reduces the runtime overhead. The DOM updates are as minimal as possible, leading to potentially better performance, especially in simpler applications or those with many small, frequent updates.
- More Predictable Performance: Direct DOM manipulation can result in more predictable performance characteristics, as there’s no additional layer (like the Virtual DOM) that needs to process changes.
3. Reactivity and State Management
The approach to reactivity and state management is a significant aspect where React and Svelte differ, affecting how developers interact with and manage the state within applications.
React
- State and Lifecycle APIs: React’s reactivity is largely managed through its state and lifecycle APIs. In class components, this involves
setState
and various lifecycle methods. In functional components, hooks likeuseState
anduseEffect
are used. - useState and useEffect:
useState
allows you to add state to functional components, whileuseEffect
is used for side effects and can replicate the behavior of lifecycle methods in class components. - Immutability and State Updates: React emphasizes immutability in state management. When updating the state, you typically create a new copy of your state with the changes, rather than modifying the existing state directly.
- External State Management Libraries: For complex applications, React often relies on external libraries like Redux or MobX for state management. These libraries provide more structured ways to manage state, especially when dealing with large and complex data flows.
Svelte
- Simplified Reactivity Model: Svelte integrates reactivity directly into its language design. This is achieved through a simpler and more intuitive model where assigning a new value to a variable is enough to trigger UI updates.
- No Special APIs for Basic Reactivity: Unlike React, Svelte does not require the use of special APIs or methods for basic reactivity. This reduces boilerplate and makes state management more straightforward.
- Auto-Subscriptions in Templates: In Svelte, you can use variables in the markup, and Svelte will automatically update the DOM when these variables change. This direct use of variables in the markup simplifies the development process.
- Built-In Stores for Cross-Component State: For more complex state management, especially when sharing state across multiple components, Svelte provides a built-in concept of stores. Stores in Svelte offer a simple and effective way to manage reactive state outside of component hierarchies.
4. Learning Curve and Complexity
The learning curve and complexity associated with React and Svelte are important considerations for developers choosing a framework, especially for those new to web development or transitioning from another technology.
React
- Conceptual Overhead: Learning React involves understanding a range of concepts, including JSX, components, state and props, lifecycle methods, and hooks. This can be challenging for beginners.
- Hooks and Advanced Features: The introduction of hooks in React added a powerful new way to use features like state and side effects in functional components. However, mastering hooks and their rules (like the rules of hooks) adds to the learning curve.
- Ecosystem Complexity: React’s ecosystem is vast, with numerous libraries for state management (e.g., Redux, MobX), routing (e.g., React Router), and more. While this offers flexibility, it also means that developers need to make more decisions and learn additional tools.
- Frequent Updates and Best Practices: React’s environment is dynamic, with frequent updates and evolving best practices. Keeping up with these changes requires ongoing learning and adaptation.
Svelte
- Simplicity and Less Boilerplate: Svelte is designed to be simpler and more intuitive, especially for beginners. It requires less boilerplate code, making it easier to start building applications without mastering a lot of concepts upfront.
- Integrated Reactivity Model: Svelte’s reactivity model is straightforward, eliminating the need to learn separate APIs for managing state and side effects. This reduces the initial complexity for new developers.
- Clearer Syntax and Semantics: Svelte’s syntax and semantics are closer to vanilla HTML, CSS, and JavaScript, which can be more approachable for those with basic front-end development knowledge.
- Smaller Ecosystem: While Svelte’s ecosystem is growing, it’s currently smaller and less complex than React’s. This can be a double-edged sword: it’s easier to grasp but offers fewer choices and resources.
5. Performance
The performance characteristics of React and Svelte are key factors in their adoption and use, especially in scenarios where efficiency and speed are critical.
React
- Good Baseline Performance: React generally provides good performance for a wide range of applications. Its efficient update algorithm and the use of the Virtual DOM help in minimizing unnecessary DOM manipulations, which are expensive in terms of performance.
- Virtual DOM Overhead: While the Virtual DOM is efficient in many scenarios, it can introduce overhead, especially in very complex applications with frequent updates. The process of diffing and updating the Virtual DOM itself consumes resources.
- Need for Performance Tuning: In more complex applications, developers often need to optimize performance proactively. Techniques like memoization, shouldComponentUpdate, React.memo, and careful state management are used to control re-rendering and reduce the workload.
- Server-Side Rendering (SSR): React supports SSR, which can improve the performance of initial page loads and is beneficial for SEO. However, implementing SSR can be complex and might require additional configuration and optimization.
Svelte
- Compile-Time Optimizations: Svelte’s unique approach of being a compiler allows it to optimize the code during the build phase. It generates highly efficient imperative code that updates the DOM directly.
- No Virtual DOM: Svelte does away with the Virtual DOM entirely. Direct DOM manipulation based on state changes tends to be more efficient, especially in applications where changes are frequent and localized.
- Generally Better Performance in Smaller Applications: In smaller applications or in scenarios with many small, frequent updates, Svelte’s approach can offer significant performance benefits. The lack of Virtual DOM overhead and the optimized compiled code generally result in faster updates.
- Less Need for Manual Optimization: Due to its design, Svelte often requires less manual performance tuning compared to React. The framework’s compiler is designed to produce optimal code out-of-the-box.
6. Community and Ecosystem
The community and ecosystem surrounding a technology are crucial, impacting the availability of resources, support, and the overall development experience. React and Svelte present contrasting pictures in this regard:
React
- Vast and Established Community: React, being one of the most popular JavaScript libraries for building user interfaces, has a vast and well-established community. This large community translates into a wealth of shared knowledge, resources, and support.
- Extensive Library and Tool Ecosystem: The React ecosystem is replete with libraries, tools, extensions, and integrations. This abundance of resources offers developers various options for almost every need, from state management (like Redux) to routing (like React Router) and UI components (like Material-UI).
- Rich Learning Resources: Due to its popularity, there are extensive learning resources available for React, including official documentation, tutorials, courses, forums, and community support channels. This makes it easier for new developers to learn and for experienced developers to find solutions to complex problems.
- Frequent Updates and Innovations: The large and active community also drives continuous improvements and innovations in the React ecosystem. Developers benefit from regular updates, new tools, and evolving best practices.
Svelte
- Growing Community: Svelte has a growing community of developers and enthusiasts. Although smaller compared to React’s, it’s known for its friendliness and supportive nature, which can be particularly encouraging for newcomers.
- Emerging Ecosystem of Tools and Libraries: The Svelte ecosystem is less extensive but is rapidly growing. New tools, libraries, and resources are continuously being developed, although the range is not as vast as in React’s ecosystem.
- Quality over Quantity: The Svelte community, while smaller, often focuses on quality and simplicity. The tools and libraries in the Svelte ecosystem tend to align with the framework’s philosophy of simplicity and ease of use.
- Accessible Learning Resources: There are increasing learning materials available for Svelte, including official documentation, tutorials, and community guides. These resources are generally straightforward and easy to follow, reflecting the framework’s emphasis on simplicity.
React vs. Svelte: Which Is the Best for you?
When deciding between React and Svelte for your project, the “best” choice depends on various factors tailored to your specific needs, preferences, and circumstances. Here’s a breakdown to help you determine which might be the best fit for you:
Project Requirements and Scale
- Complex, Large-Scale Applications: If you’re building a large-scale application with complex state management and dynamic content, React’s robust ecosystem, and wide array of libraries and tools make it a strong contender.
- Smaller to Medium-Scale Projects: For simpler applications, personal projects, or when rapid development is a priority, Svelte’s streamlined approach can lead to faster development and potentially better performance.
Team Expertise and Learning Curve
- Existing React Knowledge: If you or your team are already experienced with React, it might be more efficient to leverage that expertise. The learning and transition cost to Svelte might outweigh its benefits in this case.
- New to Web Development: For those new to web development or without a strong preference, Svelte’s easier learning curve and simpler syntax could provide a smoother entry point.
Performance Considerations
- Performance Optimization: If performance, especially initial load time and responsiveness, is a high priority, Svelte’s compile-time optimizations and direct DOM updates offer advantages.
- Large, Interactive UIs: For applications with extensive and complex UI interactions, React’s Virtual DOM and state management patterns provide a proven framework for managing reactivity and performance.
Community Support and Resources
- Need for Community and Library Support: React’s vast community and extensive range of libraries and tools are invaluable for troubleshooting, learning, and extending the capabilities of your application.
- Preference for a Simpler Ecosystem: If a smaller, but growing, community and a less complex ecosystem are appealing to you, Svelte’s environment might be more suitable.
Long-Term Maintenance and Evolution
- Long-Term Maintenance: Consider the long-term aspects of your project. React’s stability and widespread adoption might offer more predictability for long-term projects.
- Adopting New Technologies: If your project or organization values early adoption of new, potentially more efficient technologies, Svelte’s innovative approach could be a draw.
FAQS
1. What are the key differences between Svelte and React?
- Svelte is a compiler that generates efficient JavaScript code for creating UIs, whereas React is a JavaScript library that uses a Virtual DOM to manage UI rendering. Svelte offers a more straightforward approach with less boilerplate, while React provides a robust ecosystem with extensive library support.
2. Is Svelte easier to learn than React?
- Generally, Svelte is considered easier to learn for beginners due to its simpler syntax and less boilerplate code. React, while powerful, has a steeper learning curve due to its extensive ecosystem and more complex concepts like hooks and state management.
3. How does performance in Svelte compare to React?
- Svelte often has a performance edge, especially in smaller applications, due to its compile-time optimizations and absence of a Virtual DOM. React is performant but may require additional optimization in complex applications.
4. Can Svelte be a good alternative to React for large applications?
- While Svelte is growing in capability, React is generally preferred for large-scale applications due to its mature ecosystem, extensive community support, and proven track record in handling complex projects.
5. What are the job market prospects for Svelte compared to React?
- The job market for React is currently larger and more established compared to Svelte. However, Svelte’s growing popularity may lead to increased job opportunities in the future.