JSON, an acronym for JavaScript Object Notation, is a streamlined data interchange format. Its simplicity and clarity make it a favorite among developers and machines alike. Here’s why:
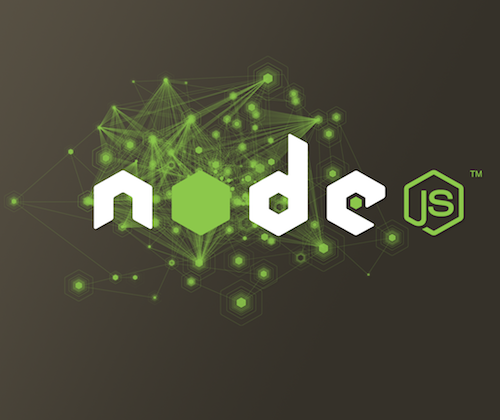
Human-Friendly: JSON’s structure is intuitive and easy to comprehend, making it straightforward for developers to read and write.
Machine-Optimized: Its design ensures that machines can efficiently parse and generate it.
Versatility: JSON’s widespread adoption means it’s the go-to format for data storage, data transmission between a server and a client, and powering web APIs.
In web development, especially with Node.js, understanding how to use JSON effectively is crucial.
This article aims to comprehensively understand how to read and write JSON files in Node.js. By the end, you’ll have the knowledge and techniques to handle JSON files effectively within a Node.js environment.
Node.js: Its Essence and Affinity with JSON
Node.js is an open-source, cross-platform JavaScript runtime environment allowing developers to execute JavaScript code outside a browser. Here’s why it’s a top pick for handling JSON files:
- Efficiency: Node.js operates on the V8 engine, renowned for its speed, making JSON file operations swift and seamless.
- Non-blocking I/O: Node.js’s asynchronous nature ensures that operations, like reading or writing to a file, don’t stall other tasks.
- Native JSON Support: Since JSON is a subset of JavaScript, Node.js natively supports JSON, making parsing and stringifying operations straightforward.
Use Cases in Node.js with JSON Files
- Configuration Files: Storing configuration settings in a JSON file, such as database connection details.
- Data Storage: Using JSON files as a lightweight database for small-scale applications.
- API Responses: Sending data to the client in JSON format when building RESTful APIs.
- Package Management: The package.json file in Node.js projects contains metadata about the project and its dependencies.
Tips and Best Practices
- Schema Validation: Always validate the structure of your JSON data to ensure consistency and avoid potential errors.
- Error Handling: Implement robust error handling, especially when reading from or writing to files, to manage unexpected issues gracefully.
- Pretty Printing: When writing JSON data, consider using the ‘pretty print’ option to make it more readable, especially during development.
- Backup Regularly: If using JSON for data storage, ensure regular backups to prevent data loss.
- Unit Testing: Incorporate unit tests to verify the correct reading and writing operations of your JSON files, ensuring the reliability of your application.
What Is JSON and Why Does It Matter?
JSON, or JavaScript Object Notation, is officially defined as a lightweight data interchange format. Its design is rooted in a subset of the JavaScript language, but its utility extends beyond just JavaScript. It’s a text format that is both human-readable and machine-friendly, making it a universal choice for data representation in modern computing.
Several experts in the field of web and software development have weighed in on the importance of JSON. Here’s a synthesis of their perspectives:
- Universality: While JSON originates from JavaScript, its format is so generic that it can be easily adopted by almost any programming language. This universality makes it a preferred choice for data interchange across diverse platforms and languages.
- Simplicity: Experts often laud JSON for its straightforwardness. Unlike other formats, JSON’s structure is intuitive, making it easy for developers to grasp and utilize.
- Flexibility: JSON’s schema-less nature means the data structure can be altered without significant disruptions. This flexibility is particularly beneficial in dynamic environments where data requirements might evolve.
- Compatibility: JSON is a compatible format in the web services and APIs age. Its lightweight nature ensures quick data transfers and its widespread adoption means that most modern web services support it natively.
Applications of JSON in the Modern Tech Landscape
- Data Storage: JSON’s readability makes it an excellent choice for configuration files or lightweight databases.
- Data Exchange: Its compatibility and lightweight nature make JSON the go-to format for data interchange between servers and clients.
- Web APIs: RESTful services, which dominate the modern web, predominantly use JSON to transmit data, given its ease of parsing and generation.
Use Cases for Reading and Writing JSON Files in Node.js
Node.js, with its non-blocking I/O and event-driven architecture, is a perfect match for handling JSON files. Let’s delve into some common use cases based on official documentation and insights from experts in the field.
1. Configuration Files
- Description: JSON files are often used to store configuration settings for applications. This includes database credentials, API keys, environment-specific settings, and more.
- Benefits:
- Security: By externalizing configuration, you can avoid hardcoding sensitive information directly into the code, reducing potential security risks.
- Portability: Configuration files can be easily swapped or modified without changing the application’s core code, making it more adaptable to different environments.
- Maintainability: Centralizing configuration settings in a JSON file simplifies updates and ensures consistency across the application.
- Customization: Allows developers to tailor user experiences based on different configurations without altering the main application logic.
2. Data Exchange
- Description: JSON is the de facto standard for data interchange on the web. In Node.js, it’s common to read from or write to JSON files when exchanging data between different systems or applications over the internet.
- Benefits:
- Interoperability: JSON’s universal format ensures diverse systems can communicate seamlessly, irrespective of the underlying technology.
- Scalability: JSON’s lightweight nature ensures efficient data transfers, making it easier to scale applications.
- Reliability: JSON parsers are available in almost every programming language, ensuring reliable data interpretation across platforms.
- Integration Simplicity: JSON reduces the complexity of integrating disparate systems, saving time and resources.
- Enhanced Analytics: JSON’s structured format facilitates better data analytics, reporting, and visualization.
3. Package Management
- Description: In Node.js projects, the package.json file is pivotal. It contains metadata about the project, such as its dependencies, scripts, and version information.
- Benefits:
- Dependency Management: Lists all the modules and versions an application depends on, ensuring consistent builds and deployments.
- Project Metadata: Provides a snapshot of the project’s details, like its version, description, and more.
- Script Automation: Allows developers to define and run scripts, streamlining development tasks.
In essence, JSON’s versatility, combined with Node.js’s capabilities, offers a robust solution for many application needs, from configuration to data interchange.
Best Practices for Reading and Writing JSON Files in Node.js
Handling JSON files in Node.js is a common task; doing it right can save you from pitfalls. Based on official documentation and insights from seasoned developers, here are some best practices to consider:
1. Leverage Built-in Modules
- Tip: Always use Node.js’s built-in fs (File System) module for reading and writing files. It’s stable, well-documented, and designed to work seamlessly with the Node.js environment.
- Benefit: Using native modules ensures compatibility and stability and reduces the need for external dependencies.
2. Implement Robust Error Handling
- Tip: Always wrap your file reading/writing operations inside try-catch blocks. This will help you catch and handle any errors during the process.
- Benefit: It provides a mechanism to gracefully handle errors, ensuring that your application remains resilient and can provide meaningful feedback to users or developers.
3. Choose Between Synchronous and Asynchronous Methods Wisely
- Tip: If you’re not worried about blocking the event loop (for instance, during a one-off script or initialization), you can use synchronous methods like fs.readFileSync() or fs.writeFileSync().
- Benefit: Synchronous methods are straightforward, resulting in cleaner, more linear code. They’re especially useful for scripts or operations where performance isn’t a primary concern.
- Tip: For operations where performance is crucial or in scenarios where you don’t want to block the event loop (like in a web server), opt for asynchronous methods like fs.readFile() or fs.writeFile().
- Benefit: Asynchronous methods ensure the Node.js event loop isn’t blocked, allowing your application to handle other tasks concurrently. This is particularly vital for applications with high concurrency requirements.
4. Validate JSON Data
- Tip: Before writing JSON data to a file, validate its structure to ensure it’s correctly formatted. Similarly, after reading JSON data, validate it before processing.
- Benefit: Ensures data integrity and reduces the risk of runtime errors due to malformed or unexpected data.
5. Use Streams for Large Files
- Tip: If you’re dealing with large JSON files, consider using streams (fs.createReadStream() or fs.createWriteStream()) to read or write data in chunks rather than loading the entire file into memory.
- Benefit: Streams enhance performance and reduce memory consumption, making your application more scalable and efficient.
6. Backup and Versioning
- Tip: Regularly backup your JSON files, especially if they contain critical data. Consider using version control systems to track changes.
- Benefit: Provides a safety net against data loss and allows for tracking and reverting changes when necessary.
Incorporating these best practices into your Node.js projects will ensure efficient, reliable, and secure handling of JSON files, setting a solid foundation for your applications.
Conclusion
Data is the lifeblood of applications, and JSON is one of its most versatile carriers. JSON, or JavaScript Object Notation, is a lightweight data-interchange format that’s human-readable and machine-friendly. Its origin in JavaScript doesn’t limit its utility; it’s a universal choice for data representation across many programming languages.
The significance of JSON is manifold:
- Universality: Its format is easily adopted by almost any programming language, making data interchange seamless across diverse platforms.
- Simplicity & Flexibility: Its intuitive structure and schema-less nature make it easy to use and adaptable to evolving data requirements.
- Compatibility: With the rise of web services and APIs, JSON’s lightweight and standardized format ensures quick, consistent data transfers.
Node.js, with its efficient and event-driven architecture, is a prime environment for handling JSON files. Whether you’re storing configuration settings, exchanging data between systems, or managing project metadata, Node.js offers synchronous and asynchronous methods to cater to different performance needs.
However, the key to harnessing the full potential of JSON in Node.js lies in adhering to best practices:
- Utilizing built-in modules for file operations.
- Implementing robust error handling.
- Based on the application’s requirements, making informed decisions between synchronous and asynchronous operations.
- Validating and ensuring data integrity.
For those eager to delve deeper into the world of JSON and Node.js, consider exploring advanced topics such as:
- JSON Schema Validation: Ensuring the structure and content of your JSON data meet specific criteria.
- Streaming Large JSON Files: Techniques for efficiently processing massive datasets.
- Optimizing JSON Storage: Exploring databases and storage solutions optimized for JSON data.