Microservices represent a modern approach to software architecture, where applications are structured as a suite of small, self-contained services. Each of these services operates independently, yet they collectively contribute to the application’s overall functionality.
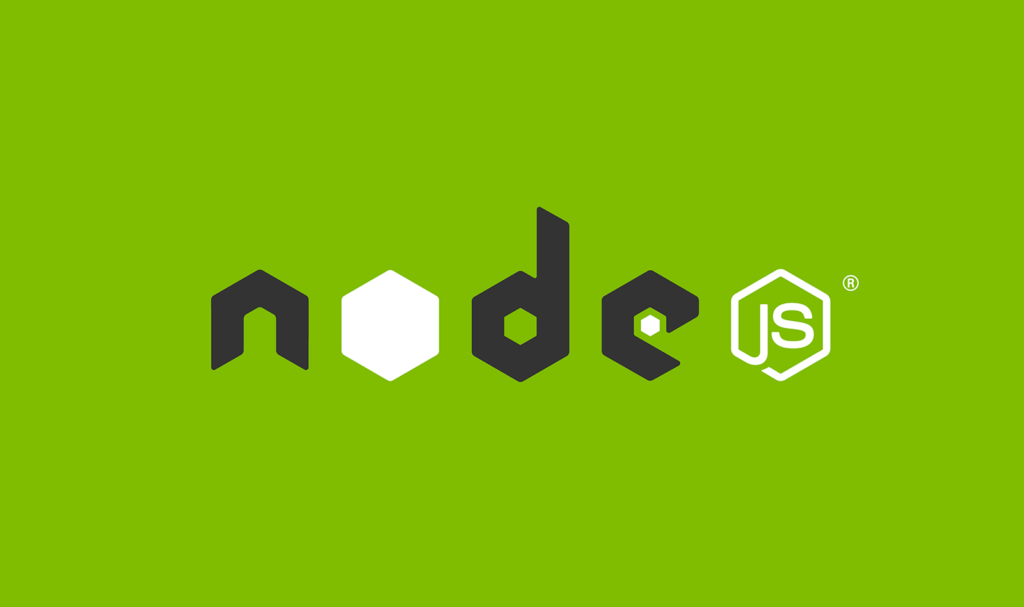
The adoption of microservices offers several advantages:
- Scalability: Microservices can be scaled individually based on demand, ensuring optimal performance without overburdening other components.
- Flexibility: With each service being independent, one can make changes without affecting the others. This modular approach allows for easier updates and enhancements.
- Maintainability: Smaller codebases are generally easier to manage and debug. Microservices can isolate and resolve issues without impacting the entire system.
- Collaboration: Development teams can work on different services simultaneously, leading to faster development cycles and reduced bottlenecks.
- Innovation and Agility: Microservices foster a culture of continuous improvement. Teams can experiment, innovate, and deploy new features rapidly, adapting to market needs with agility.
In web development, Node.js emerges as a powerful tool for building microservices, thanks to its non-blocking, event-driven architecture and vast ecosystem. As we delve deeper into this guide, we’ll explore how to harness the power of Node.js in creating efficient microservices.
Building Microservices with Node.js
This article’s primary objective is to provide readers with a clear understanding of how to construct microservices using Node.js. By the end of this guide, you’ll grasp the methodologies and strategies that ensure the effective creation of microservices with this platform.
A Deep Dive into Node.js
Node.js is an open-source, cross-platform JavaScript runtime environment allowing developers to execute JavaScript code outside a web browser. But what makes it a preferred choice for building microservices?
- Performance: Node.js operates on a non-blocking, event-driven architecture, ensuring efficient processing and handling of simultaneous operations.
- Scalability: It’s inherently designed to build scalable network applications, making it apt for microservices that require individual scaling.
- Rich Ecosystem: With npm (Node Package Manager), developers can access a vast library of modules and packages, accelerating development.
- Flexibility: Node.js doesn’t dictate any set patterns or rules, granting developers the freedom to design applications as they see fit.
Spotlight on Microservices: Use Cases and Advantages
Microservices have found their footing in a variety of applications. Here are some common scenarios:
- E-Commerce Platforms: Microservices can handle different components like user authentication, product catalog, payment gateway, and more, ensuring each component scales based on its demand.
- Streaming Services: Platforms like Netflix use microservices to manage content delivery, user preferences, and recommendations.
- Social Media Platforms: Features like feeds, notifications, chats, and friend requests can be managed as separate services.
The benefits of employing microservices in these scenarios include:
- Enhanced scalability and resilience.
- Faster time-to-market for new features.
- Independent deployment cycles.
- Improved fault isolation.
Golden Rules: Designing and Deploying Microservices with Node.js
- Design for Failure: Always assume that services can fail. Implement fallback strategies and ensure your system remains robust.
- Maintain a Consistent Communication Protocol: Whether you choose HTTP/REST, gRPC, or any other protocol, ensure consistency across all services.
- Implement Service Discovery: As services scale, they might change their location. Service discovery tools can help in locating and communicating with them.
- Centralize Logging and Monitoring: With multiple services running, centralized logging is crucial for debugging and monitoring system health.
- Secure Your Services: Implement authentication and authorization for each service. Ensure data encryption and regular security audits.
What Are Microservices and Why Do They Matter?
Microservices, often referred to as microservices architecture, is an architectural style that structures an application as a collection of small, autonomous services, modeled around a specific business domain. Let’s break this down based on official definitions and insights from industry experts:
- Official Definition: According to the Microservices.io platform, microservices are defined as “an architectural style that structures an application as a collection of loosely coupled services, which implement business capabilities.”
- Expert Opinion: Sam Newman, author of “Building Microservices,” states that microservices are “small, autonomous services that work together.”
Core Characteristics of Microservices:
- Independence: Each service in a microservices architecture is independent, meaning it can be developed, deployed, and scaled without depending on any other service.
- Communication: These services communicate with each other, often using HTTP/REST, gRPC, or message queues like RabbitMQ or Kafka.
- Single Responsibility: Following the single responsibility principle, each service is responsible for a specific functionality or business capability.
Why Do Microservices Matter?
- Scalability: One of the most significant advantages of microservices is the ability to scale individual components based on demand rather than scaling the entire application. This leads to more efficient resource utilization and cost savings.
- Flexibility and Maintainability: Given their modular nature, updates or changes can be made to a single service without affecting the entire application. This modularity also simplifies debugging and maintenance.
- Enhanced Collaboration: With microservices, development teams can work on different services simultaneously, reducing development time and fostering a culture of collaboration. Each team can focus on a specific service or feature, ensuring expertise and quality.
- Innovation and Agility: Microservices support a culture of continuous integration and continuous delivery (CI/CD). New features or improvements can be rapidly developed, tested, and deployed, allowing businesses to adapt quickly to market changes or customer demands.
- Resilience: Since each service operates independently, a failure in one service doesn’t necessarily bring down the entire application. This distributed nature enhances the overall resilience and uptime of the system.
Use Cases for Microservices and Their Benefits
Microservices have become a popular architectural choice for many industries, offering a range of benefits over traditional monolithic architectures. Let’s delve into some common use cases and their advantages, drawing from official sources and expert insights:
1. E-commerce
Use Case: E-commerce platforms are complex systems that require various functionalities, from product listing to payment processing. Microservices can be employed to manage:
- Product catalog management
- Order management and tracking
- Payment processing
- Customer reviews and ratings
- Inventory management
- User profiles and authentication
Benefits:
- Scalability: As e-commerce platforms experience fluctuating traffic (e.g., during sales or holiday seasons), microservices allow individual components, like payment processing, to scale independently, ensuring smooth user experiences.
- Customization: With microservices, it’s easier to introduce personalized recommendations or user-specific features, enhancing the shopping experience.
- Reduced Complexity: Instead of navigating a massive codebase, developers can focus on specific services, making updates or introducing new features more manageable.
- Resilience: If one service, like order tracking, faces issues, it doesn’t impact the entire platform, ensuring higher uptime and reliability.
2. Social Media
Use Case: Social media platforms are multifaceted, with features ranging from posts and comments to direct messaging and notifications. Microservices can manage:
- User authentication and profiles
- Content management (posts, images, videos)
- Messaging and chat services
- Notifications and alerts
- Friend or connection management
Benefits:
- Enhanced Security: By separating user authentication or profile management, security measures can be more robust, reducing potential data breaches.
- Flexibility: As user demands evolve, microservices allow for the rapid introduction of new features or modifications to existing ones without overhauling the entire platform.
- Interoperability: Microservices can easily integrate with third-party services or platforms, be it for advertising, analytics, or other purposes.
- Optimized Performance: With the ability to scale services based on demand, users experience reduced latency and faster load times, even during peak usage.
Expert Opinion: Chris Richardson, a recognized expert in microservices, emphasizes their role in enabling organizations to experiment, iterate, and adapt to changing requirements without the overhead of traditional architectures. This agility is evident in the use cases mentioned above, where businesses must be dynamic and responsive to user needs.
Microservices offer a modular, scalable, and efficient approach to building complex applications. Whether it’s e-commerce or social media, the benefits of adopting this architecture are manifold, leading to improved user experiences and business outcomes.
Tips and Best Practices for Building Microservices with Node.js
Building microservices with Node.js requires a strategic approach to ensure efficiency, scalability, and maintainability. Drawing from official documentation and insights from industry experts, here are some best practices to consider:
1. Define Your Architecture Based on Objectives
- Assess Your Needs: Before diving into microservices, evaluate if it’s the right fit. While microservices offer numerous benefits, they also introduce complexity. Consider factors like team expertise, existing infrastructure, and long-term goals.
- Decentralize Data Management: Each microservice should have its database to ensure loose coupling and data integrity.
2. Design Services with Architectural Best Practices
- Separation of Concerns (SoC): Ensure each microservice handles a distinct functionality or business logic. This makes the system more maintainable and reduces interdependencies.
- Single Responsibility Principle (SRP): Each service should have one reason to change. This principle ensures that the service remains focused on its specific task, making it easier to update or scale.
3. Utilize Node.js Frameworks for Efficient Implementation
- Express.js: One of the most popular minimalistic web frameworks for Node.js. It provides essential features like routing, middleware support, and a suite of plugins to extend its capabilities.
- Nest.js: A progressive Node.js framework offering a modular architecture, making it apt to build scalable microservices. It also integrates seamlessly with other libraries and tools.
4. Prioritize Testing for Robust Microservices
- Automated Testing: Use tools like Jest or Mocha for automated testing. These frameworks offer features for unit testing, integration testing, and support for mocks, stubs, and spies.
- Continuous Integration (CI): Implement a CI pipeline to test new code changes automatically. This ensures that any new addition or modification doesn’t introduce bugs or break existing functionality.
- End-to-end Testing: While unit and integration tests are crucial, they also ensure end-to-end testing to validate the entire flow of a microservice, from request to response.
5. Monitor and Log Effectively
- Centralized Logging: With multiple services running, centralized logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Graylog can help in aggregating logs, making it easier to monitor and debug issues.
- Performance Monitoring: Use tools like New Relic or Datadog to monitor the performance of your microservices, ensuring they are running optimally and efficiently.
In his book “Building Microservices,” Sam Newman emphasizes the importance of building services around business capabilities and independently deploying them. He also highlights the significance of continuous integration, testing, and monitoring in a microservices architecture.
In conclusion, while Node.js offers a robust platform for building microservices, adhering to best practices and leveraging the right tools can significantly enhance your microservices ecosystem’s efficiency, scalability, and maintainability.